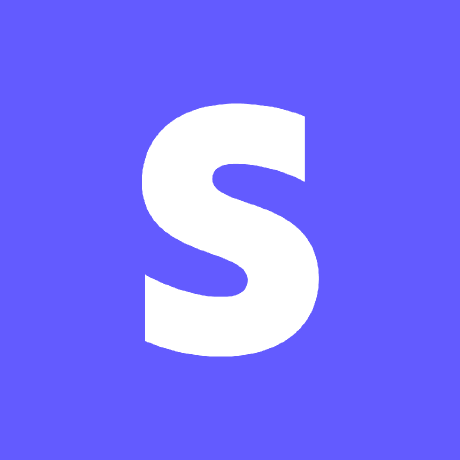
Agent Toolkit
用于将 Stripe API 集成到代理工作流中的 Python 和 TypeScript 库
Stripe 代理工具包
Stripe 代理工具包使流行的代理框架(包括 OpenAI 的 Agent SDK、LangChain、CrewAI、Vercel 的 AI SDK 和 Model Context Protocol (MCP))能够通过函数调用与 Stripe API 集成。该库并不涵盖整个 Stripe API。它支持 Python 和 TypeScript,并直接构建在 Stripe Python 和 Node SDK 之上。
下面包含了基本说明,但请参阅 Python 和 TypeScript 包以获取更多信息。
Python
安装
除非你想修改包,否则你不需要这个源代码。如果你想使用该包,请运行:
sh
pip install stripe-agent-toolkit
要求
- Python 3.11+
使用
该库需要配置你的账户的密钥,该密钥可以在 Stripe 仪表板 中找到。
python
from stripe_agent_toolkit.openai.toolkit import StripeAgentToolkit
stripe_agent_toolkit = StripeAgentToolkit(
secret_key="sk_test_...",
configuration={
"actions": {
"payment_links": {
"create": True,
},
}
},
)
该工具包适用于 OpenAI 的 Agent SDK、LangChain 和 CrewAI,并可以作为工具列表传递。例如:
python
from agents import Agent
stripe_agent = Agent(
name="Stripe Agent",
instructions="You are an expert at integrating with Stripe",
tools=stripe_agent_toolkit.get_tools()
)
OpenAI 的 Agent SDK、LangChain 和 CrewAI 的示例包含在 /examples 中。
上下文
在某些情况下,你可能希望提供一些值作为请求时的默认值。目前,account
上下文值使你能够为你的 连接账户 进行 API 调用。
python
stripe_agent_toolkit = StripeAgentToolkit(
secret_key="sk_test_...",
configuration={
"context": {
"account": "acct_123"
}
}
)
TypeScript
安装
除非你想修改包,否则你不需要这个源代码。如果你想使用该包,请运行:
npm install @stripe/agent-toolkit
要求
- Node 18+
使用
该库需要配置你的账户的密钥,该密钥可以在 Stripe 仪表板 中找到。此外,configuration
允许你指定可以使用工具包执行的操作类型。
typescript
import { StripeAgentToolkit } from "@stripe/agent-toolkit/langchain";
const stripeAgentToolkit = new StripeAgentToolkit({
secretKey: process.env.STRIPE_SECRET_KEY!,
configuration: {
actions: {
paymentLinks: {
create: true,
},
},
},
});
工具
该工具包适用于 LangChain 和 Vercel 的 AI SDK,并可以作为工具列表传递。例如:
typescript
import { AgentExecutor, createStructuredChatAgent } from "langchain/agents";
const tools = stripeAgentToolkit.getTools();
const agent = await createStructuredChatAgent({
llm,
tools,
prompt,
});
const agentExecutor = new AgentExecutor({
agent,
tools,
});
上下文
在某些情况下,你可能希望提供一些值作为请求时的默认值。目前,account
上下文值使你能够为你的 连接账户 进行 API 调用。
typescript
const stripeAgentToolkit = new StripeAgentToolkit({
secretKey: process.env.STRIPE_SECRET_KEY!,
configuration: {
context: {
account: "acct_123",
},
},
});
计量计费
对于 Vercel 的 AI SDK,你可以使用中间件来提交使用情况的计费事件。所需的是客户 ID 和输入/输出计量器。
typescript
import { StripeAgentToolkit } from "@stripe/agent-toolkit/ai-sdk";
import { openai } from "@ai-sdk/openai";
import {
generateText,
experimental_wrapLanguageModel as wrapLanguageModel,
} from "ai";
const stripeAgentToolkit = new StripeAgentToolkit({
secretKey: process.env.STRIPE_SECRET_KEY!,
configuration: {
actions: {
paymentLinks: {
create: true,
},
},
},
});
const model = wrapLanguageModel({
model: openai("gpt-4o"),
middleware: stripeAgentToolkit.middleware({
billing: {
customer: "cus_123",
meters: {
input: "input_tokens",
output: "output_tokens",
},
},
}),
});
模型上下文协议
Stripe 代理工具包还支持 模型上下文协议 (MCP)。
要使用 npx 运行 Stripe MCP 服务器,请使用以下命令:
bash
npx -y @stripe/mcp --tools=all --api-key=YOUR_STRIPE_SECRET_KEY
将 YOUR_STRIPE_SECRET_KEY
替换为你的实际 Stripe 密钥。或者,你可以在环境变量中设置 STRIPE_SECRET_KEY。
或者,你可以设置自己的 MCP 服务器。例如:
typescript
import { StripeAgentToolkit } from "@stripe/agent-toolkit/modelcontextprotocol";
import { StdioServerTransport } from "@modelcontextprotocol/sdk/server/stdio.js";
const server = new StripeAgentToolkit({
secretKey: process.env.STRIPE_SECRET_KEY!,
configuration: {
actions: {
paymentLinks: {
create: true,
},
products: {
create: true,
},
prices: {
create: true,
},
},
},
});
async function main() {
const transport = new StdioServerTransport();
await server.connect(transport);
console.error("Stripe MCP Server running on stdio");
}
main().catch((error) => {
console.error("Fatal error in main():", error);
process.exit(1);
});
支持的 API 方法
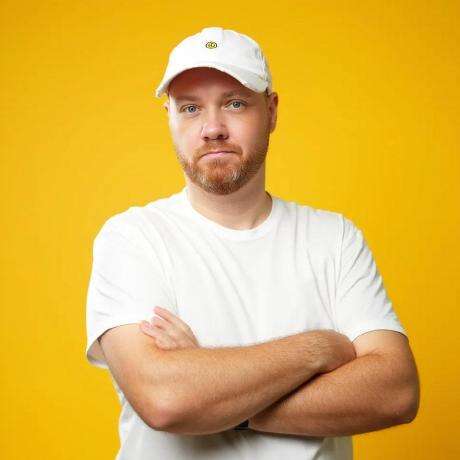
4o Mini Search Mcp
提供通过轻量级服务器连接到 OpenAI 或 OpenRouter.ai 的搜索启用模型的网页搜索功能,设置要求极简。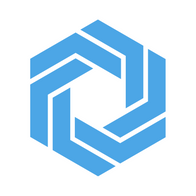
Agora Mcp
Agora MCP 服务器,可在数千家在线商店中搜索和购买商品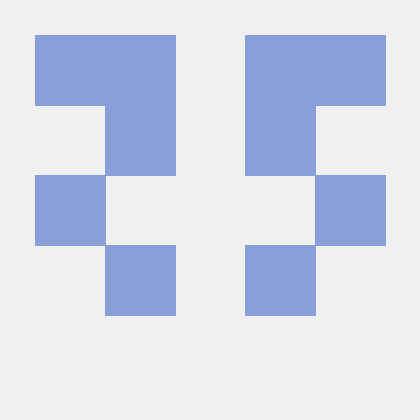
Ai Agent Marketplace Index Mcp
MCP 服务器用于 DeepNLP 的 AI 代理市场索引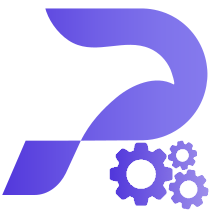